The Positive Influence of the Using Declaration
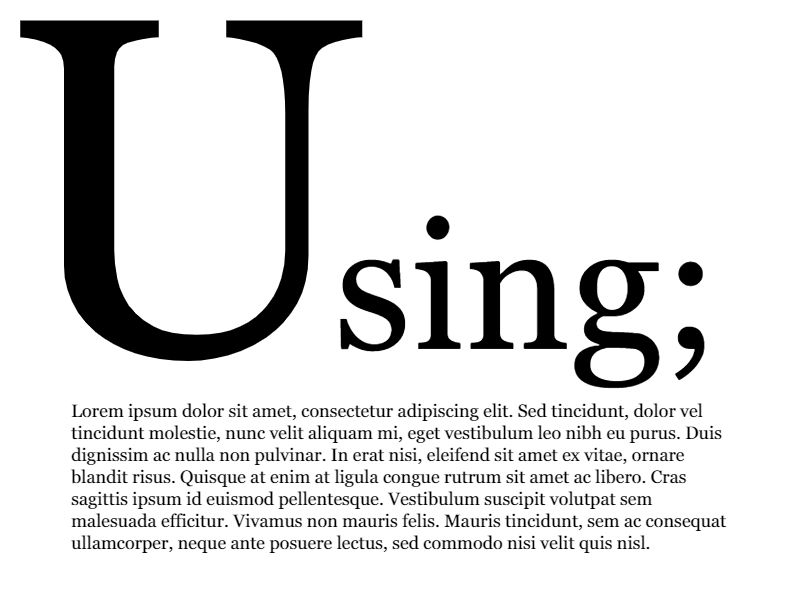
Software engineering is challenging at times. Sometimes it can feel like riding a runaway train. No matter your level of discipline these moments of challenge will happen, often when you least expect it. That is why I promote the patterns and practices I do: to keep the train on the rails as much as possible.
One of the practices I recently added was in C# to switch from using statements to using declarations. Initially my thought was to avoid this new language feature, but after some consideration I decided that it can be a positive influence. I wanted to mention early on about my evolution with this new language feature, so those of you who (rightfully) consider it a potentially dangerous option will know that I did not immediately warm to its use.
What exactly did I eventually see in the using declaration? Well, to better highlight that let’s look at the using statement first. What I aim to point out is an inherent weakness in the using statement construct - and then contrast that with the using declaration to show why it can be a positive influence on my software practice.
Using Statement
The using statement essentially wraps a scope in a dispose command (AutoClosable for those more familiar with Java). Here is an example using statement very similar to the general pattern I see with my clients. It’s actually shorter than the average, but more on that later.
I’m going to assume you grok the above, but basically it acquires a disposable object and then uses that object to potentially add to its “channels” projection. When the using statement exits the disposable object is disposed. I’ve seen thousands of blocks like this - as I’m sure have you. Nothing strange or fancy here.
Nothing strange or fancy, but there is an inherent weakness. As I alluded to earlier, the average using statement block length is longer than the above example. And while vertical length does not necessarily indicate vertical complexity, let’s be real: there is a correlation between block length and having multiple responsibilities.
So why the tendency towards long using statement blocks? It’s right there in my description of what a using statement is:
The using statement essentially wraps a scope in a dispose command
As a developer we implicitly know the using statement block is a command with the bonus of disposing a disposable resource. The block encourages us to do whatever needs doing before the resources are disposed of. Regardless of how long the block, the end result is the same: the resource is disposed of. This out of sight, out of mind can cause us to be less aware of - or ignore completely - that a resource is “open”. The effect is that we code as if there is no resource, and that can open the door to doing more than a single thing.
Now let’s contrast that with the using declaration and see how this weakness of encouraging long block length is flipped on its head.
Using Declaration
The main difference between the using statement and the using declaration is that the latter does not introduce a new scope. The current scope is used. In practice that means the current method - although there is nothing stopping you from creating a sub-scope within the method (then you might as well use the using statement).
Let’s take a look at your average using declaration.
Here the disposable object is disposed of when HandleEvent exits. And this is where the using declaration can have a positive influence: it encourages your method to be short and singularly focused. Why?
Typically you want your disposable object to be disposed of as close as possible to where the object was created. There’s two reasons for that: 1) It is more likely the resource will be held for the shortest appropriate duration (short block can correlate to short duration); 2) It is more readable. I recall a quote from Clean Code:
Indeed, the ratio of time spent reading versus writing is well over 10 to 1. We are constantly reading old code as part of the effort to write new code...making it easy to read makes it easier to write.Robert C. Martin
As developers we implicitly know that a using declaration is somewhat of a loaded gun. If we are not careful the disposable object will stick around longer than desirable (or safe). Thus we are influenced to keep the containing method as short and singularly focused as possible.
If you have not tried the using declaration yet then try it - but in production code where the stakes are real. I predict you will feel a heightened sense of responsibility for the open resource. I wonder what happens to your methods (and your classes) as a result.
Conclusion
Okay, clearly this post is about a much bigger picture than the little ol’ using declaration. It is about letting go of things that have a negative influence on your development practice. It is about putting positive influences at the core.
Here are some infrequently discussed things that can have a positive influence on your development practice. They may seem minor, but truly I have found that it is the aggregation of many minor influences that actually move the dial.
Zen Mode
If your editor has a zen mode then use it. If not then hide all toolbars, tool windows, etc. I often see people coding in a fraction of their screen size, and rarely using all the cruft that is thus wasting space. If you need a tool then learn the hotkey to open and close it.
Hotkeys
Speaking of hotkeys, learn them and use them. Taking your hands off the keyboard leaves an opening for a distraction.
Multiple Monitors
Use as many monitors as you can. Reserve one monitor for communication tools (e.g., Slack, email) and keep its notifications away from your (coding) work at hand.
Roadmap
Maintain an hourly and daily ROADMAP summarizing work to be done, work in progress, and work completed. You know you’re going to have to have this information for timesheets and/or ticketing systems anyway - so get in the practice and watch how this influences you.
Schedule Later
Get comfortable saying “not right now but I should have time later”. Developers are constantly bombarded with demands for their time: meetings, PRs, support, etc. The less context switching you do, the more focused your code will be.
Don’t Surf
Let go of browsing personal stuff (e.g., YouTube, Instagram, Facebook) when you could be working.
Write Tests
I know it’s extra work, but I have found it saves me time in the end because my code “just works” when I’m done. The time spent finding and fixing issues ends up being far more than the time to write tests.
Write Good Code
Good code begets more good code. It’s perhaps the most positive influence I have found.
This is just a short list as this post is in danger of becoming too long. You get the gist: keep an eye out for things that positively influence your development; keep those close, shed the rest.
I hope some or all of this post has a positive influence on your development practice.